Set up EDB for Power Integrity Analysis#
This example shows how to set up the electronics database (EDB) for power integrity analysis from a single configuration file.
Import the required packages#
[1]:
import json
[2]:
import os
import tempfile
from pyaedt import Hfss3dLayout
from pyaedt.downloads import download_file
from pyedb import Edb
AEDT_VERSION = "2024.1"
NG_MODE = False
Download the example PCB data.
[3]:
temp_folder = tempfile.TemporaryDirectory(suffix=".ansys")
download_file(source="touchstone", name="GRM32_DC0V_25degC_series.s2p", destination=temp_folder.name)
file_edb = download_file(source="edb/ANSYS-HSD_V1.aedb", destination=temp_folder.name)
Load example layout#
[4]:
edbapp = Edb(file_edb, edbversion=AEDT_VERSION)
PyAEDT INFO: Logger is initialized in EDB.
PyAEDT INFO: legacy v0.21.1
PyAEDT INFO: Python version 3.10.11 (tags/v3.10.11:7d4cc5a, Apr 5 2023, 00:38:17) [MSC v.1929 64 bit (AMD64)]
PyAEDT INFO: Database ANSYS-HSD_V1.aedb Opened in 2024.1
PyAEDT INFO: Cell main Opened
PyAEDT INFO: Builder was initialized.
PyAEDT INFO: EDB initialized.
Create an empty dictionary to host all configurations#
[5]:
cfg = dict()
Assign S-parameter model to capactitors.#
Set S-parameter library path.
[6]:
cfg["general"] = {"s_parameter_library": os.path.join(temp_folder.name, "touchstone")}
Assign the S-parameter model.
Keywords
name. Name of the S-parameter model in AEDT.
component_definition. Known as component part number of part name.
file_path. Touchstone file or full path to the touchstone file.
apply_to_all. When set to True, assign the S-parameter model to all components share the same component_definition. When set to False, Only components in “components” are assigned.
components. when apply_to_all=False, components in the list are assigned an S-parameter model. When apply_to_all=False, components in the list are NOT assigned.
reference_net. Reference net of the S-parameter model.
[7]:
cfg["s_parameters"] = [
{
"name": "GRM32_DC0V_25degC_series",
"component_definition": "CAPC0603X33X15LL03T05",
"file_path": "GRM32_DC0V_25degC_series.s2p",
"apply_to_all": False,
"components": ["C110", "C206"],
"reference_net": "GND",
}
]
Define ports#
Create a circuit port between power and ground nets.
Keywords
name. Name of the port.
reference_desinator.
type. Type of the port. Supported types are ‘ciruict’, ‘coax’.
positive_terminal. Positive terminal of the port. Supported types are ‘net’, ‘pin’, ‘pin_group’, ‘coordinates’.
negative_terminal. Positive terminal of the port. Supported types are ‘net’, ‘pin’, ‘pin_group’, ‘coordinates’.
[8]:
cfg["ports"] = [
{
"name": "port1",
"reference_designator": "U1",
"type": "circuit",
"positive_terminal": {"net": "1V0"},
"negative_terminal": {"net": "GND"},
}
]
Define SIwave SYZ analysis setup#
Keywords
name. Name of the setup.
type. Type of the analysis setup. Supported types are ‘siwave_ac’, ‘siwave_dc’, ‘hfss’.
pi_slider_position. PI slider position. Supported values are from ‘0’, ‘1’, ‘2’. 0:speed, 1:balanced, 2:accuracy.
freq_sweep. List of frequency sweeps.
name. Name of the sweep.
type. Type of the sweep. Supported types are ‘interpolation’, ‘discrete’, ‘broadband’.
frequencies. Frequency distribution.
distribution. Supported distributions are ‘linear_count’, ‘linear_scale’, ‘log_scale’.
start. Start frequency. Example, 1e6, “1MHz”.
stop. Stop frequency. Example, 1e9, “1GHz”.
increment.
[9]:
cfg["setups"] = [
{
"name": "siwave_syz",
"type": "siwave_ac",
"pi_slider_position": 1,
"freq_sweep": [
{
"name": "Sweep1",
"type": "interpolation",
"frequencies": [{"distribution": "log_scale", "start": 1e6, "stop": 1e9, "increment": 20}],
}
],
}
]
Define Cutout#
Keywords
signal_list. List of nets to be kept after cutout.
reference_list. List of nets as reference planes.
extent_type. Supported extend types are ‘Conforming’, ‘ConvexHull’, ‘Bounding’. For optional input arguments, refer to method pyedb.Edb.cutout()
[10]:
cfg["operations"] = {
"cutout": {
"signal_list": ["1V0"],
"reference_list": ["GND"],
"extent_type": "ConvexHull",
}
}
Write configuration into as json file#
[11]:
file_json = os.path.join(temp_folder.name, "edb_configuration.json")
with open(file_json, "w") as f:
json.dump(cfg, f, indent=4, ensure_ascii=False)
Import configuration into example layout#
[12]:
edbapp.configuration.load(config_file=file_json)
[12]:
<pyedb.configuration.cfg_data.CfgData at 0x18023e15320>
Apply configuration to EDB.
[13]:
edbapp.configuration.run()
PyAEDT INFO: Cutout Multithread started.
PyAEDT INFO: Net clean up Elapsed time: 0m 6sec
PyAEDT INFO: Expanded Net Polygon Creation Elapsed time: 0m 1sec
PyAEDT INFO: Padstack Instances removal completed. 1310 instances removed. Elapsed time: 0m 1sec
PyAEDT INFO: Primitives cleanup completed. 265 primitives deleted. Elapsed time: 0m 4sec
PyAEDT INFO: Deleted 702 additional components
PyAEDT INFO: Cutout completed. Elapsed time: 0m 12sec
[13]:
True
Save and close EDB.
[14]:
edbapp.save()
edbapp.close()
[14]:
True
The configured EDB file is saved in a temp folder.
[15]:
print(temp_folder.name)
C:\Users\ansys\AppData\Local\Temp\tmpo7nofv2d.ansys
Load edb into HFSS 3D Layout.#
[16]:
h3d = Hfss3dLayout(edbapp.edbpath, version=AEDT_VERSION, non_graphical=NG_MODE, new_desktop=True)
PyAEDT INFO: Initializing new Desktop session.
PyAEDT INFO: StdOut is enabled
PyAEDT INFO: Log on file is enabled
PyAEDT INFO: Log on Desktop Message Manager is enabled
PyAEDT INFO: Debug logger is disabled. PyAEDT methods will not be logged.
PyAEDT INFO: Launching PyAEDT outside AEDT with gRPC plugin.
PyAEDT INFO: New AEDT session is starting on gRPC port 50172
PyAEDT INFO: AEDT installation Path C:\Program Files\AnsysEM\v241\Win64
PyAEDT INFO: Ansoft.ElectronicsDesktop.2024.1 version started with process ID 2716.
PyAEDT INFO: pyaedt v0.9.9
PyAEDT INFO: Python version 3.10.11 (tags/v3.10.11:7d4cc5a, Apr 5 2023, 00:38:17) [MSC v.1929 64 bit (AMD64)]
PyAEDT INFO: AEDT 2024.1.0 Build Date 2023-11-27 22:16:18
PyAEDT INFO: EDB folder C:\Users\ansys\AppData\Local\Temp\tmpo7nofv2d.ansys\edb/ANSYS-HSD_V1.aedb has been imported to project ANSYS-HSD_V1
PyAEDT INFO: Active Design set to main
PyAEDT INFO: Aedt Objects correctly read
Analyze#
[17]:
h3d.analyze()
PyAEDT INFO: Key Desktop/ActiveDSOConfigurations/HFSS 3D Layout Design correctly changed.
PyAEDT INFO: Solving all design setups.
PyAEDT INFO: Key Desktop/ActiveDSOConfigurations/HFSS 3D Layout Design correctly changed.
PyAEDT INFO: Design setup None solved correctly in 0.0h 0.0m 29.0s
[17]:
True
Plot impedance#
[18]:
solutions = h3d.post.get_solution_data(expressions="Z(port1,port1)")
solutions.plot()
C:\actions-runner\_work\pyedb\pyedb\.venv\lib\site-packages\pyaedt\generic\plot.py:55: UserWarning: The PyVista module is required to run some functionalities of PostProcess.
Install with
pip install pyvista
Requires CPython.
warnings.warn(
PyAEDT INFO: Parsing C:/Users/ansys/AppData/Local/Temp/tmpo7nofv2d.ansys/edb/ANSYS-HSD_V1.aedt.
PyAEDT INFO: File C:/Users/ansys/AppData/Local/Temp/tmpo7nofv2d.ansys/edb/ANSYS-HSD_V1.aedt correctly loaded. Elapsed time: 0m 0sec
PyAEDT INFO: aedt file load time 0.0624997615814209
PyAEDT INFO: Loading Modeler.
PyAEDT INFO: Modeler loaded.
PyAEDT INFO: EDB loaded.
PyAEDT INFO: Layers loaded.
PyAEDT INFO: Primitives loaded.
PyAEDT INFO: Modeler class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: PostProcessor class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: Post class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: Solution Data Correctly Loaded.
[18]:
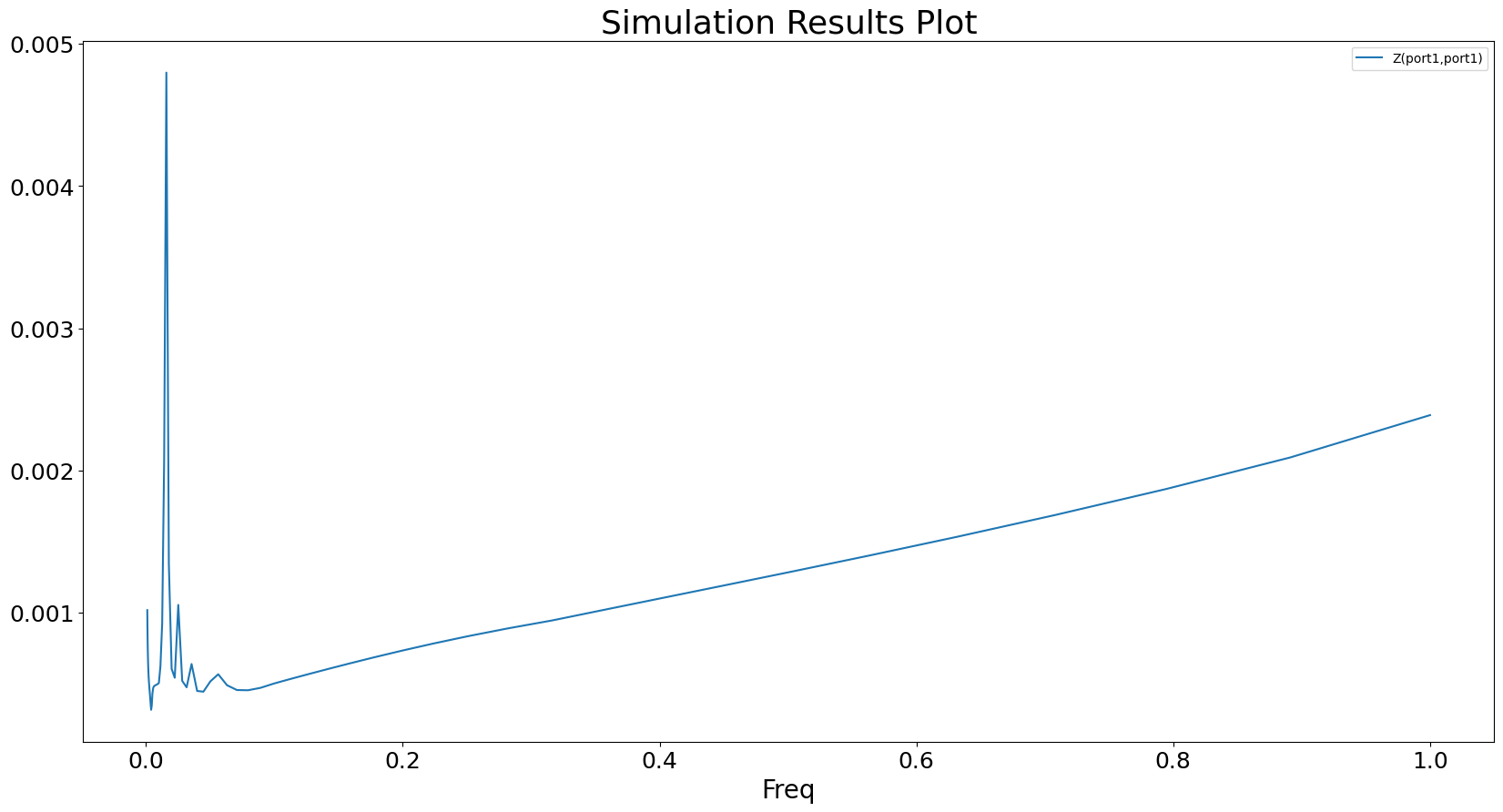
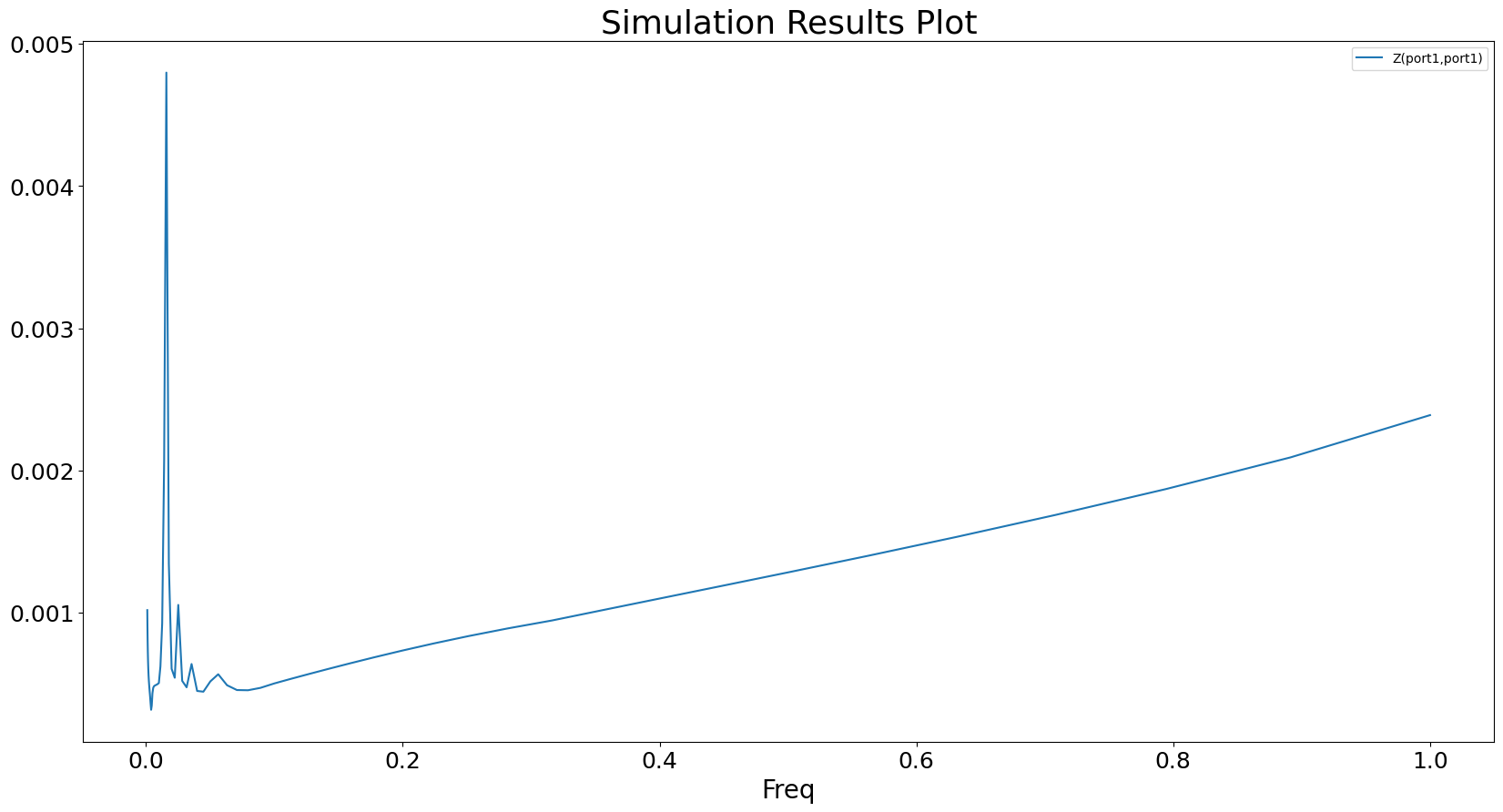
Shut Down Electronics Desktop#
[19]:
h3d.close_desktop()
PyAEDT INFO: Desktop has been released and closed.
[19]:
True
All project files are saved in the folder temp_file.dir
. If you’ve run this example as a Jupyter notbook you can retrieve those project files.