Note
Go to the end to download the full example code.
EDB: IPC2581 export#
This example shows how you can use PyEDB to export an IPC2581 file.
Perform required imports#
Perform required imports, which includes importing a section.
import os
import pyedb
from pyedb.generic.general_methods import (
generate_unique_folder_name,
generate_unique_name,
)
from pyedb.misc.downloads import download_file
Download file#
Download the AEDB file and copy it in the temporary folder.
temp_folder = generate_unique_folder_name()
targetfile = download_file("edb/ANSYS-HSD_V1.aedb", destination=temp_folder)
ipc2581_file = os.path.join(temp_folder, "Ansys_Hsd.xml")
print(targetfile)
C:\Users\ansys\AppData\Local\Temp\pyedb_prj_9MU\edb/ANSYS-HSD_V1.aedb
Launch EDB#
Launch the pyedb.Edb
class, using EDB 2023 R2 and SI units.
edb = pyedb.Edb(edbpath=targetfile, edbversion="2024.1")
Parametrize net#
Parametrize a net.
edb.modeler.parametrize_trace_width("A0_N", parameter_name=generate_unique_name("Par"), variable_value="0.4321mm")
True
Cutout#
Create a cutout.
signal_list = []
for net in edb.nets.netlist:
if "PCIe" in net:
signal_list.append(net)
power_list = ["GND"]
edb.cutout(
signal_list=signal_list,
reference_list=power_list,
extent_type="ConvexHull",
expansion_size=0.002,
use_round_corner=False,
number_of_threads=4,
remove_single_pin_components=True,
use_pyaedt_extent_computing=True,
extent_defeature=0,
)
[[0.010419265503311216, 0.04198192227822067], [0.010460827148201247, 0.04177297777950732], [0.010508394252349874, 0.04160868358656615], [0.011378955665958661, 0.039345987891904084], [0.011481104429354762, 0.03914889754796645], [0.011613614198034717, 0.03895058246243907], [0.011738277022225034, 0.03879868020873362], [0.013993114009542158, 0.03654384060066575], [0.021723676859529008, 0.02881328031371329], [0.021875579015058783, 0.028688617457459438], [0.022142311790681247, 0.02851039231475559], [0.022498901023239357, 0.028361153409415505], [0.0501081997161374, 0.022658443118289424], [0.050115389883144214, 0.022656985466385306], [0.05024405459822067, 0.022631392463311216], [0.05043961576046106, 0.022612131370000003], [0.056204646439538916, 0.022612131370000003], [0.05640020760177925, 0.02263139246331121], [0.056580308047582455, 0.022667216669352046], [0.05668897431368785, 0.022695247080691455], [0.06716106242493838, 0.02603293367394693], [0.06722884511408374, 0.026057258249349364], [0.07246895216307161, 0.028153570607566375], [0.07280808931839765, 0.02837720713327149], [0.07316535186536138, 0.02873446968023509], [0.07342865686053635, 0.029190527309764774], [0.07354078107549526, 0.029608980576750453], [0.07357500163, 0.029868911494369585], [0.07357500163, 0.030067860929093797], [0.07357500153781718, 0.030068290307276504], [0.07357236964157587, 0.03619784264682524], [0.07350964817259248, 0.036547407037203675], [0.07286766739910838, 0.03828106548337578], [0.07276100213239857, 0.03849009301684572], [0.07262496955638041, 0.038693680154024815], [0.07250030658211681, 0.03884558242767533], [0.06923416782767555, 0.04211172118211658], [0.0690822655540256, 0.04223638415637981], [0.06891128756716963, 0.042350627994751505], [0.0687750106459916, 0.042427029682916874], [0.06457857080039528, 0.04436633092671103], [0.06455011001328882, 0.044378949674346774], [0.03731543182981512, 0.05595023588676457], [0.037118962655707244, 0.056010807858163314], [0.0368930736417795, 0.056055739976688765], [0.03669751247953899, 0.056075001070000005], [0.016627488820461125, 0.056075001070000005], [0.01643192765822088, 0.05605573997668881], [0.016113662838625083, 0.055992433167969397], [0.015749686071864895, 0.05584166905478863], [0.015479874185974789, 0.055661386516380106], [0.015327971912324545, 0.05553672354211668], [0.013513280597883254, 0.05372203222767539], [0.013388617623619748, 0.05357012995402501], [0.013291281391863361, 0.05342445598866224], [0.013242137524933176, 0.05334272651686749], [0.011218869861076723, 0.049583054764648575], [0.01113324211279165, 0.049367262126055834], [0.011088479294635446, 0.04920020501440689], [0.011079768081486809, 0.04916517771943696], [0.010453155795310887, 0.04643625086590004], [0.010447006513881935, 0.04640754503977299], [0.010419265503311206, 0.04626808156177926], [0.01040000441, 0.04607252039953892], [0.01040000441, 0.04217748344046106]]
Plot cutout#
Plot cutout before exporting to IPC2581 file.
edb.nets.plot(None, None, color_by_net=True)
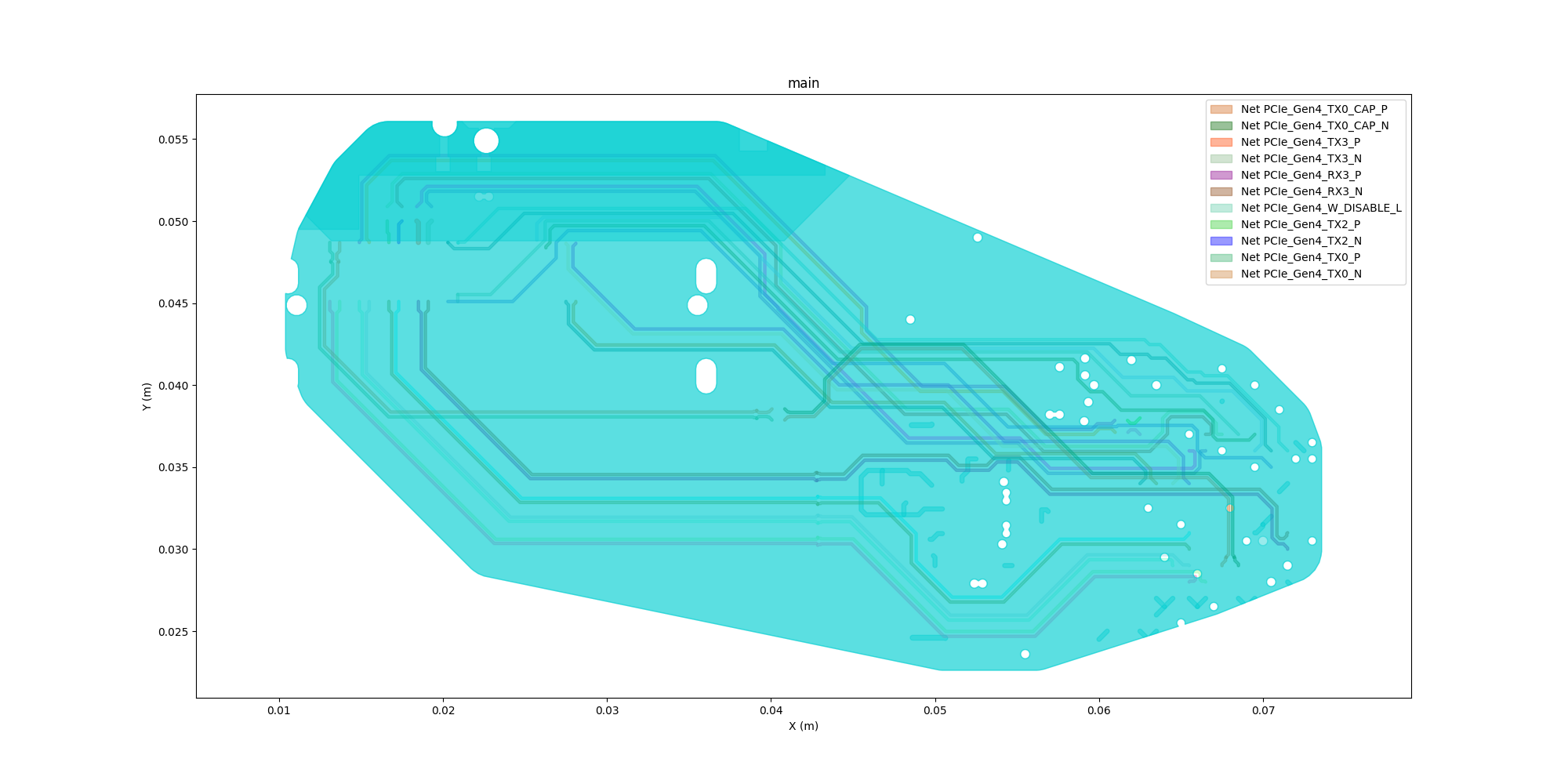
Create IPC2581 file#
Create the IPC2581 file.
edb.export_to_ipc2581(ipc2581_file, "inch")
print("IPC2581 File has been saved to {}".format(ipc2581_file))
IPC2581 File has been saved to C:\Users\ansys\AppData\Local\Temp\pyedb_prj_9MU\Ansys_Hsd.xml
Close EDB#
Close EDB.
edb.close_edb()
True
Total running time of the script: (0 minutes 9.943 seconds)